Laravel 11 is shaping up to be one of the most exciting and transformative updates to the popular PHP framework. Packed with significant changes, new features, and thoughtful refinements, Laravel 11 promises to enhance developer experience and streamline application development. Let’s explore what makes Laravel 11 a game changer.
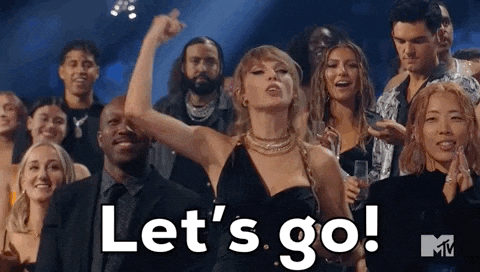
1. Slim Skeleton: A Minimalistic Approach
One of the most significant changes in Laravel 11 is the introduction of a more minimalistic application skeleton. The default folder structure for new Laravel projects has been simplified, focusing on essentials:
app/
├── Http/
│ └── Controllers/
│ └── Controller.php
├── Models/
│ └── User.php
└── Providers/
└── AppServiceProvider.php
bootstrap/
├── app.php
└── providers.php
config/
...
Key Changes:
- Centralized Configuration: Routes, middleware, and exceptions are now registered in
bootstrap/app.php
.
- Removed Folders: The
app/Console
,app/Exceptions
, andapp/Http/Middleware
directories have been removed.
Example of bootstrap/app.php
:
use Illuminate\Foundation\Application;
use Illuminate\Foundation\Configuration\Exceptions;
use Illuminate\Foundation\Configuration\Middleware;
return Application::configure(basePath: dirname(__DIR__))
->withRouting(
web: __DIR__.'/../routes/web.php',
commands: __DIR__.'/../routes/console.php',
health: '/up',
)
->withMiddleware(function (Middleware $middleware) {
//
})
->withExceptions(function (Exceptions $exceptions) {
//
})->create();
The routes
folder has also been cleaned up, removing routes/channel.php
and routes/api.php
by default.
2. Removed Config Files
Laravel 11 introduces a slimmer configuration setup, removing files like config/broadcasting.php
, config/cors.php
, and others. However, these can still be published manually using:
php artisan config:publish
or
php artisan config:publish --all
This change reduces clutter while maintaining flexibility.
3. Optional API and Broadcasting
Routes and tools like Sanctum and broadcasting are now optional. Developers can install them using the following commands:
Install API Scaffolding:
php artisan install:api
Install Broadcasting:
php artisan install:broadcast
These commands dynamically register routes and install necessary packages only when required.
4. New Defaults: Pest and SQLite
- Default Database: SQLite is the default database for local development, reducing setup friction.
- Testing Framework: Pest is now the default testing framework, chosen for its simplicity and elegance.
5. New Artisan Commands
Laravel 11 introduces new make:
commands to generate enums, interfaces, and classes:
php artisan make:enum
php artisan make:class
php artisan make:interface
These commands simplify the creation of common structures and enhance developer productivity.
6. Health Check Endpoint
A new health check route (/up
) is included by default. This route provides a simple way to monitor application health and performance.
Example:
return Application::configure(basePath: dirname(__DIR__))
->withRouting(
health: '/up',
)
->create();
The /up
endpoint fires a DiagnosingHealth
event and can display diagnostic information or custom responses.
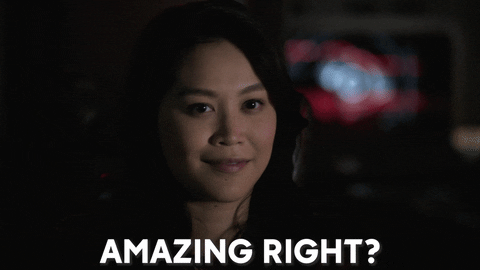
7. Dumpable Trait
Debugging becomes easier with the new Dumpable
trait. It enables inline usage of dd()
and dump()
methods in framework classes and user-defined classes.
Example:
use Illuminate\Support\Traits\Dumpable;
class Address
{
use Dumpable;
// ...
}
$address = new Address;
$address->setThis()->dd()->setThat();
8. Limit Eager Loading
Developers can now limit eagerly loaded records natively without relying on external packages:
$users = User::with(['posts' => function ($query) {
$query->latest()->limit(10);
}])->get();
9. New casts()
Method
The new casts()
method allows for defining model casts as a protected method, providing more flexibility and organization.
Example:
protected function casts(): array
{
return [
'email_verified_at' => 'datetime',
'password' => 'hashed',
];
}
This method is prioritized over the $casts
property.
10. Once Memoization Method
The once()
function ensures a callable is executed only once per instance, caching the result for subsequent calls:
class User extends Model
{
public function stats(): array
{
return once(function () {
// Expensive operations
return $stats;
});
}
}
This enhances performance for operations requiring repeated calls within the same instance.
Laravel 11 introduces numerous improvements that streamline workflows, enhance debugging, and simplify application configuration. Whether it’s the slim skeleton, new artisan commands, or enhanced testing defaults, this version solidifies Laravel’s position as a cutting-edge framework for modern PHP development.
Feel free to browse the official documentation : https://laravel.com/docs/11.x/upgrade