Introduction
Tired of apps that crumble under pressure? With FastAPI, you can build robust, high-performance applications that scale seamlessly. FastAPI is a modern, fast (high-performance) web framework for Python that makes it easy to create APIs that can handle millions of requests per second. Whether you’re building a startup MVP or a production-grade solution, FastAPI provides the tools you need for scalability.
In this article, we’ll explore how to build a scalable school management application using FastAPI, complete with code examples and best practices.
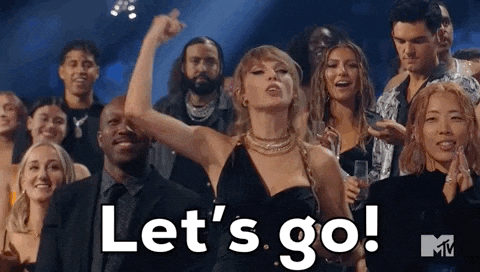
Why Scalability Matters
Scalability ensures your application can handle increased demand without compromising performance. For a school management app, this could mean handling thousands of students, teachers, and administrative staff accessing the system simultaneously. FastAPI’s asynchronous capabilities, type hints, and support for modern Python features make it an ideal choice for creating scalable applications.
1. FastAPI Fundamentals
Setting Up a Basic FastAPI Application
FastAPI’s simplicity is unmatched. Here’s how to set up a basic application:
from fastapi import FastAPI
app = FastAPI()
@app.get("/")
def read_root():
return {"message": "Welcome to the School Management System!"}
@app.get("/students/{student_id}")
def read_student(student_id: int):
return {"student_id": student_id, "details": "Student details here."}
Run the application with:
uvicorn main:app --reload
Visit http://127.0.0.1:8000/docs
to access the interactive API documentation, thanks to OpenAPI.
2. Building the Foundation
Project Structure
Organize your FastAPI project for maintainability and scalability:
school_management/
├── app/
│ ├── main.py
│ ├── routers/
│ │ ├── students.py
│ │ ├── teachers.py
│ │ └── courses.py
│ ├── models/
│ ├── schemas/
│ └── services/
├── requirements.txt
├── Dockerfile
└── README.md
Database Layer
Use SQLAlchemy or Tortoise-ORM for database operations. Here’s an example with SQLAlchemy:
Install Dependencies
pip install sqlalchemy asyncpg databases
Configure Database
from sqlalchemy import create_engine, Column, Integer, String, ForeignKey
from sqlalchemy.ext.declarative import declarative_base
from sqlalchemy.orm import sessionmaker, relationship
DATABASE_URL = "postgresql+asyncpg://user:password@localhost/school_db"
Base = declarative_base()
engine = create_engine(DATABASE_URL)
SessionLocal = sessionmaker(autocommit=False, autoflush=False, bind=engine)
def get_db():
db = SessionLocal()
try:
yield db
finally:
db.close()
class Student(Base):
__tablename__ = "students"
id = Column(Integer, primary_key=True, index=True)
name = Column(String, index=True)
age = Column(Integer)
courses = relationship("Enrollment", back_populates="student")
class Course(Base):
__tablename__ = "courses"
id = Column(Integer, primary_key=True, index=True)
title = Column(String, index=True)
description = Column(String)
enrollments = relationship("Enrollment", back_populates="course")
class Enrollment(Base):
__tablename__ = "enrollments"
id = Column(Integer, primary_key=True, index=True)
student_id = Column(Integer, ForeignKey("students.id"))
course_id = Column(Integer, ForeignKey("courses.id"))
student = relationship("Student", back_populates="courses")
course = relationship("Course", back_populates="enrollments")
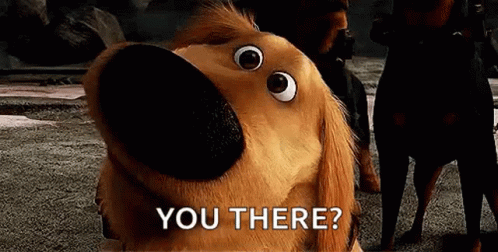
3. Implementing Scalability Principles
a) Asynchronous Operations
FastAPI’s core is built on asynchronous programming, making it perfect for handling high-concurrency scenarios.
Example: Fetching Student Data Asynchronously
from fastapi import FastAPI, Depends
from sqlalchemy.ext.asyncio import AsyncSession
from app.database import get_async_session
from app.models import Student
app = FastAPI()
@app.get("/students")
async def get_students(db: AsyncSession = Depends(get_async_session)):
result = await db.execute("SELECT * FROM students")
return result.fetchall()
b) Caching
Use Redis to cache frequently accessed data like class schedules or student details.
Install Redis
pip install aioredis
Caching Example
import aioredis
from fastapi import FastAPI, Depends
app = FastAPI()
redis = aioredis.from_url("redis://localhost")
@app.get("/cached-students")
async def get_cached_students():
data = await redis.get("students")
if not data:
data = {"students": ["John Doe", "Jane Smith"]}
await redis.set("students", str(data))
return data
c) Load Balancing
Distribute traffic across multiple instances of your FastAPI app using NGINX:
NGINX Configuration
upstream school_app {
server 127.0.0.1:8000;
server 127.0.0.1:8001;
}
server {
listen 80;
location / {
proxy_pass http://school_app;
}
}
4. Scaling Beyond Code
a) Containerization with Docker
Dockerfile
FROM python:3.10
WORKDIR /app
COPY requirements.txt .
RUN pip install -r requirements.txt
COPY . .
CMD ["uvicorn", "app.main:app", "--host", "0.0.0.0", "--port", "8000"]
b) Orchestrating with Kubernetes
Deploy your FastAPI school management app on Kubernetes for automatic scaling:
Kubernetes Deployment
apiVersion: apps/v1
kind: Deployment
metadata:
name: school-app-deployment
spec:
replicas: 3
selector:
matchLabels:
app: school-app
template:
metadata:
labels:
app: school-app
spec:
containers:
- name: school-app
image: school-app-image
ports:
- containerPort: 8000
5. Real-World Application
For our school management app, you can include features like:
- Notifications: Real-time updates for teachers, students, and parents using WebSockets.
- Student Enrollment: Register students for courses.
- Attendance Tracking: Keep records of student attendance.
- Grade Management: Manage and retrieve grades.
6. Tips and Best Practices
- Rate-Limiting: Use middleware to prevent abuse.
- Reusable Components: Modularize your code to make it reusable.
- Documentation: Leverage FastAPI’s built-in OpenAPI docs.
Conclusion
FastAPI’s modern architecture makes building scalable applications straightforward and enjoyable. By applying these principles to a school management system, you’ll create a platform that can handle the growing demands of educational institutions.
Start building your scalable school management application with FastAPI today, and let us know about your journey in the comments!
Feel free to leave a comment to give me your opinion on the article?